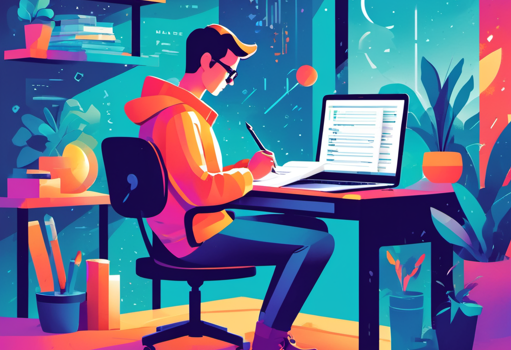
How to get into Software Engineering
I have recently been asked by a couple friends and some non technical colleagues what the best way to get into software engineering is. I wanted to give them good advice, so I wrote some notes, did a bit of introspection, and even consulted a couple of my engineer teammates. I came up with what I consider a pretty solid set of tips, practices, and general insights that I can offer people looking to enter the field. On the advice of my coworker (we will call him MO for anonymity), I have decided to put it all together here in a post that I can refer people to in the future.
This post will be broken down into sections: Motivation, School, Things to Learn, Industry tools to Know, Process, Resources and Advice from a Senior.
Motivation
So why would someone even want to become a software engineer? It's no secret that software engineering has ballooned in popularity due to the massive salaries. It's not abnormal for 22 year olds to make solid 6 figure incomes in their first full time job. For some people this is the only motivation they need to embark on the software journey. Others are intrigued by the promise of intellectually challenging problems, remote work, or working with computers all day. There are many reasons to want to get into the field and I am not here to say that any are better than any others, I will just provide some advice based on the anecdotal experience of me, and some of my much more experienced colleages.
Are you in school?
Yes -> Great! Pay attention and enjoy the ride!No -> Go to school!
Now before you click off, let me explain... I don't think that college is the only way to be successful as a software engineer. I have met engineers with no college degrees who easily outshine engineers with degrees. I do think that college is a great way to have the main peices of information and theory you need shoved down your throat though. I think if you have the ability to go to college you should, but if you can't I still think you should go to school.
When I say "school" I am not necessarily saying you should go to a building with teachers and pay tuition and all that, but I think you should treat your learning like school. You should have a lesson plan (preferably set by someone who knows more than you), you should hold yourself to deadlines and homework. The best way to learn and get better is to treat the process as something important and keep yourself accountable. On top of that, I think you are likely to learn the things that you need more quickly if you follow someone else's curriculum. I will talk more about specific resources later, but don't try to do it all on your own in the dark, take help from the people trying to offer it.
Things to Learn
So what should an aspiring software engineer learn? Here is my comprehensive list. I won't go into detail, these are just concepts you should keep track of during your journery and check off as you get to them. In general order from basic/most important to harder/least important
Basic Concepts
- Control Flow - loops, conditionals, functions
- Types - strings, integers, arrays, etc. and how to manipulate them
Data Structures
- Classes/Objects/Structs
- Arrays/Lists
- Maps/Sets - Including the hashed versions
- Heaps/Queues/Stacks
- Graphs
Algorithms
- Basic Array Iteration
- Recursion
- Binary Search
- Sorting - understanding sorting algorithms is a great foot in the door to understanding the basics of most algorithms
- BFS, DFS - Breadth First Search, Depth First Search
- Graph Traversal
Industry Standard Technologies
- Code Editor - Choose a code editor and get comfortable with it, make sure the editor is not your limitting factor
- Git/Github - This is a must, all your code should be version controlled for practice and to look back at. Additionally, learn how to use the CLI
- Debugger - Many people (usually me) can get buy with simple `printf()` debugging, but using a debugger is a useful skill that can set you apart in interviews
- Terminal - Get comfortable with using your terminal (preferably bash or zsh), it will make you more efficient and can help set you apart among inexperienced folks
- Docker - A widely used technology, and a must for developing and deploying more complex applications easily
This list is far from everything, but I think it is a good start to get your competent and grow as a developer. You do NOT need to wait to know all of these things to start applying for jobs, they are each just an arrow in your quiver as you learn to write software.
Process
So here is my general process for learning and growing as a developer. This is a life long journey that, once you start, will probably never end, but as you continue you
will become more competent and start to find set your own path.
The first step is to learn the basics. Choose a language to start with, I personally think JavaScript has the most versitality and is a good starting point. Start doing some lessons,
checkout the resources section for this. MAKE SOMETHING! I know people who only read books or theory, and they are nowhere near as successful or talented as the
engineers I know who do practical work. Make something that does something. It does not need to be a successful start up, or even a new idea. Make a TODO app, or a blog or anything,
just prove to yourself and potential employers that you are capable of doing things, and learn along the way. Absorb Content. Whether it is a book, a blog post,
Youtube, classes, or anything else, iterate on what you know. Improving 1% each day will net massive gains over longer periods of time. Repeat. This is an ongoing
process which will require many iterations to make real progress with. You won't understand what you are doing and you will get discouraged and confused. Just follow the steps and
keep iterating.
An additional step is to reach out to people. I would not be writing this if not for the people who reached out to me, and I would never have gotten into this industry if I had not gone out on a limb and reached out to some of my connections. Most people are willing to talk with you, offer knowledge or glance at your resume. You are never too unqualified to apply or speak to someone, the worst that happens is you get rejected 🤷♂️.
Resources
I am including here a brief list of resources that I like. There are hundreds more out there about every topic, the important thing is to explore and learn. I want to note that the people I refer to below are people I respect and enjoy in terms of software content, that endorsement does not necessarily extend beyond that.
- freeCodeCamp - A great resource for learning different aspects of software and exposure to real life projects.
- LeetCode - Infamous in the SWE community, LeetCode is a must for learning and practicing algorithms, I touch on the how a bit in the next section.
- Youtube - There are many great channels to learn from on Youtube, a few I enjoy are Fireship, ThePrimeagen, JDH, TJ DeVries, and many more. Get out there and consume some content.
- Twitch - I personally love consuming programming content on Twitch, there are many great channels, but my favorite at the moment are ThePrimeagen, Teej_dv, Melkey.
Additional Advice From a Senior Engineer
The prior mentioned MO gave me some things to add here that helped him and people he knows on his journey, so I will include them here.
- Narrow your focus. Learning one technology or language or stack is a much more efficient way to learn. No one expects you to know everything, but once you can understand a technology well it is much easier to translate that knowledge to other technologies.
- Treat LeetCode like a real project. Create a repo for your LeetCode problems, write the solutions as a program and have test files to run against your program, this is invaluable experience it teaches your about testing, project development, test driven development, and will expose you to more technologies.
- Create architecture diagrams. We don't have time to go into them here, but MO proposes treating your personal projects like real business projects and creating architecture diagrams you can present and explain in interviews. He explains that they are a great way to show interviewers what the project really consists of and how well you understand it.
- Get Exposure. Keep your ear to the ground, read articles, listen to podcasts, etc. You don't need to understand every technology, but it is super helpful to have a feel for the industry.
Hope this was helpful, I wish you good luck and happy hacking ✌️